Automating Web Testing with Playwright: An Example Testing a Job Listing Application
Automated testing has become an essential part of the software development process as web applications evolve and become more complex. With the rise of single-page applications and asynchronous events, it has become increasingly difficult to test web applications reliably and efficiently. This is where tools like Playwright come in. Playwright is designed to handle the newer approach in web development, making it easier for testers to automate the testing process and ensure that web applications work as intended. This tool is similar to other popular automation frameworks such as Selenium, Puppeteer and Cypress.
What is playwright?
Playwright is a popular end-to-end testing framework that allows developers to automate browser tests across different platforms and browsers. It is built on top of the WebKit and Chromium engines, making it highly reliable and efficient. With Playwright, developers can quickly automate browser tests, perform automation tasks, and test web pages in different browsers and platforms.
Playwright is a relatively new tool that Microsoft introduced in 20201 . It is built on top of the WebKit and Chromium engines and provides a high-level API for controlling web browsers. Playwright is designed to be fast, reliable, and easy to use.
Key Features of Playwright
- Cross-browser testing: Playwright allows you to run your tests on multiple browsers, such as Chrome, Firefox, and Safari, ensuring that your website works on all the major browsers.
- Automated Browser Context Switching: Playwright can handle the asynchronous nature of modern web applications, making it easy to automate tasks such as switching between tabs and windows.
- Element Selection & Interaction: Playwright has a built-in element selector, which makes it easy to interact with elements on the page, such as buttons, links, and input fields.
- Test Generation & Inspection: Playwright has a Test Generator and Test Inspector feature, which allows you to generate test scripts and inspect page elements.
- Test Fixtures: Playwright allows you to define reusable test fixtures, which makes it easy to set up and tear down test environments.
- Screenshots & Videos on Test Failures: Playwright allows you to capture screenshots and videos of your tests when they fail, which makes it easy to debug and fix test failures.
- Auto-Waiting Mechanism: Playwright has an auto-waiting mechanism that automatically waits for elements to appear, eliminating manual waits and making tests more reliable.
- Retries: Playwright allows you to retry failed tests, which can help to reduce flaky tests and improve test reliability.
Advantages of using Playwright
- Playwright is built on WebKit and Chromium, making it highly reliable and efficient.
- Playwright allows developers to automate browser tests across different platforms and browsers, making it easy to test web pages on different devices and browsers.
- Playwright's API is simple and easy to use, making it accessible for developers of all skill levels.
- Playwright's ability to work with multiple browsers allows developers to test web pages on different browsers and platforms, making identifying and fixing cross-browser compatibility issues easier.
- Playwright supports headless mode, allowing developers to run browser tests on servers or in the background without using a GUI.
One of the most significant advantages of Playwright is its ability to test web applications across multiple browsers and devices. This is especially useful for organisations that must ensure their web applications are compatible with different browsers and devices.
Live example to create a test script with Playwright
We have used playwright to automate testing our careers pages. The problem we will be solving is ensuring that when a user clicks on the "Apply now" button on a job listing, the job code is automatically populated in the application form.
The test case we will use is selecting a job listing from the careers page randomly, clicking on the "Apply now" button, and then checking if the job code is correctly populated in the application form.
Test Case: Verify Job Code Auto-Population on Career Page
Test Steps:
- Open the careers page on the website "zyxware.com" by navigating to the URL "https://www.zyxware.com/careers"
- Select a random job listing and job code by locating the specific class and using a random function to select an element from the list of job listings.
- Click on the "apply now" button on the selected job listing by locating the element with the role "link" and the name "Apply now."
- Check if the job code is auto-populated in the form by locating the input field with the specific id and comparing its value to the job code obtained in step 2.
Expected Result: The job code obtained in step 2 should match the value in the "edit-job-id" field, indicating that the job code was successfully auto-populated in the form.
Solution
We use Playwright to automate browser tests to solve this problem. Playwright allows us to automate browser tests and perform tasks like clicking buttons and filling out forms.
We use the following script to automate the testing: careers.spec.js
const {
test,
expect
} = require('@playwright/test');
test('Test Job Code', async ({
browser,
page
}) => {
// Open the page abc.com/careers
await page.goto('https://www.zyxware.com/careers');
// Find a random teaser item from the list of teaser items
const teaserItems = page.locator('.career-openings-page-row.clearfix.views-row article');
const count = await teaserItems.count();
const randomTeaserItem = teaserItems.nth(Math.floor(Math.random() * count));
const jobLink = randomTeaserItem.locator('a');
const jobCodeElement = await randomTeaserItem.locator('.field.field--field-job-code');
await expect(jobCodeElement).toHaveCount(1);
const jobCode = await jobCodeElement.innerText();
// Click on the apply now button on the teaser item's page
await jobLink.click();
const applyNowButton = await page.locator('#block-careerapplyblock').getByRole('link', {
name: 'Apply now'
});
const popupPromise = page.waitForEvent('popup');
await applyNowButton.click();
const popup = await popupPromise;
await popup.waitForLoadState();
// Check if the job code input field has the correct value
const jobCodeInput = await popup.locator("#edit-job-id");
const jobCodeValue = await jobCodeInput.inputValue();
//Check whether the job code is auto populated in the form.
await expect(jobCodeValue).toEqual(jobCode);
});
To execute this test in multiple browsers, create a config file with the following content: playwright.config.js
// playwright.config.js
// @ts-check
const { devices } = require('@playwright/test');
/** @type {import('@playwright/test').PlaywrightTestConfig} */
const config = {
projects: [
{
name: 'chromium',
use: { ...devices['Desktop Chrome'] },
},
{
name: 'firefox',
use: { ...devices['Desktop Firefox'] },
},
{
name: 'webkit',
use: { ...devices['Desktop Safari'] },
},
],
};
module.exports = config;
Once you have both careers.spec.js and playwright.config.js in a folder, you can execute the following command to execute the test in Chrome, Firefox and Safari.
npx playwright test --reporter=html
The detailed report will be available with the command
npx playwright show-report
Result
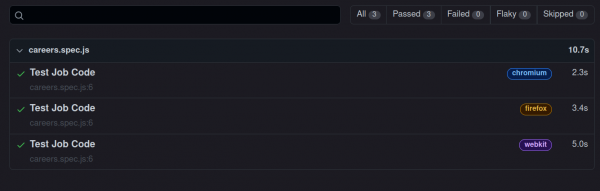
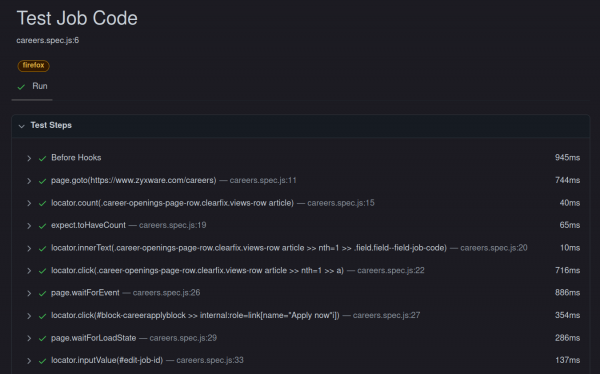
You can run playwright in debug mode to get a full walkthrough of the process.
[With inputs from Vimal Joseph.]